# Internationalization
The I18n module allows the internationalization and update of strings in the editor UI
WARNING
This guide is referring to GrapesJS v0.15.9 or higher
The module was added recently so we're open to receive support in translating strings in other languages. Your help will be much appreciated!
# Configuration
By default, the editor includes only the English language, if you need other languages you have to import them manually. Note: The language code is defined in the ISO 639-1 (opens new window) standard.
import grapesjs from 'grapesjs';
import it from 'grapesjs/locale/it';
import tr from 'grapesjs/locale/tr';
const editor = grapesjs.init({
...
i18n: {
// locale: 'en', // default locale
// detectLocale: true, // by default, the editor will detect the language
// localeFallback: 'en', // default fallback
messages: { it, tr },
}
});
Now the editor will be translated in Italian for those browsers which default language is Italian (by default detectLocale
option is enabled)
# Update strings
If you need to change some default language strings you can easily update them by using I18n API.
To find the correct path of the string you can check the en
locale file (opens new window) and follow its inner path inside the locale object.
Let's say we want to update the default message of the empty state in Style Manager when no elements are selected.
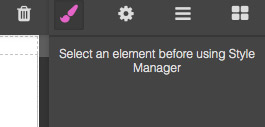
From the en
locale file you can see it by following the path below
{
...
styleManager: {
empty: 'Select an element before using Style Manager',
...
},
...
}
So now to update it you'll do this
editor.I18n.addMessages({
en: {
// indicate the locale to update
styleManager: {
empty: 'New empty state message',
},
},
});
Even if the UI shows correctly the updated message, we highly suggest to do all the API calls wrapped in a plugin
const myPlugin = editor => {
editor.I18n.addMessages({ ... });
// ...
}
grapesjs.init({
// ...
plugins: [myPlugin],
});
# Generated strings
Not all the strings are indicated in the en
local file as some of them can be generated from id
s, name
s, etc.
If you look back at the styleManager
path from the en
file you'll notice the empty properties
key
...
styleManager: {
...
properties: {
// float: 'Float',
},
...
},
...
This object is used to translate property names inside StyleManager, so if you need, for instance, to change the auto-generated names for the margin
properties
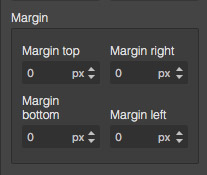
you'd this
editor.I18n.addMessages({
en: {
styleManager: {
properties: {
// The key is the property name (or id)
'margin-top': 'Top',
'margin-right': 'Right',
'margin-left': 'Left',
'margin-bottom': 'Bottom',
},
},
},
});
# Adding new language
If you want to support GrapesJS by adding a new language to our repository all you need to do is to follow steps below:
- First of all, be sure to check the language file in
src/i18n/locale
(opens new window) doesn't exist already - Open a new issue (opens new window) to avoid overlap with other contributors. To be sure, check also no one else has opened already an issue for the same language
- Start a new branch from
dev
- Copy (in the same folder) and rename the
en
locale file (opens new window) to the name of your language of choice (be sure to be compliant to ISO 639-1 (opens new window)) - Now you can start translating strings
- By following comments you'll probably notice that some keys are not indicated (eg.
styleManager.properties
), for the reference you can check other locale files - Once you've done, you can create a new Pull Request on GitHub from your branch to
dev
by making also a reference to your issue in order to close it automatically once it's merged (your PR message should containCloses #1234
where 1234 is the issue ID)
# Plugin development
WARNING
This section is dedicated only to plugin developers and can also be skipped in case you use grapesjs-cli (opens new window) to init your plugin project
If you're developing a plugin for GrapesJS and you need to support some string localization or simply change the default one, we recommend the following structure.
plugin-dir
- package.json
- README.md
- ...
- src
- index.js
- locale // create the locale folder in your src
- en.js // All default strings should be placed here
For your plugin specific strings, place them under the plugin name key
// src/locale/en.js
export default {
'grapesjs-plugin-name': {
yourKey: 'Your value',
},
};
In your index.js
use the en.js
file and add i18n
option to allow import of other local files
// src/index.js
import en from 'locale/en';
export default (editor, opts = {}) => {
const options = {
i18n: {},
// ...
...opts,
};
// ...
editor.I18n.addMessages({
en,
...options.i18n,
});
};
The next step would be to compile your locale files into <rootDir>/locale
directory to make them easily accessible by your users. This folder could be ignored in your git repository be should be deployd to the npm registry
WARNING
Remember that you can skip all these long steps and init your project with grapesjs-cli (opens new window). This will create all the necessary folders/files/commands for you (during init
command this step is flagged true
by default and we recommend to keep it even in case the i18n is not required in your project)
At the end, your plugin users will be able to import other locale files (if they exist) in this way
import grapesjs from 'grapesjs';
// Import from your plugin
import yourPlugin from 'grapesjs-your-plugin';
import ch from 'grapesjs-your-plugin/locale/ch';
import fr from 'grapesjs-your-plugin/locale/fr';
const editor = grapesjs.init({
...
plugins: [ yourPlugin ],
pluginsOpts: {
[yourPlugin]: {
i18n: { ch, fr }
}
}
});